014.Bitmap
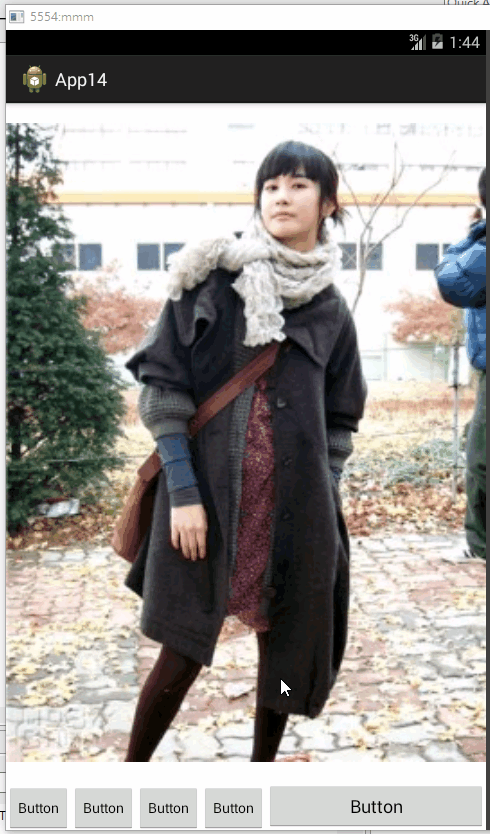
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/LinearLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="${relativePackage}.${activityClass}" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/ic_launcher" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<Button
android:id="@+id/button1"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onClick1"
android:text="Button" />
<Button
android:id="@+id/button2"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onClick2"
android:text="Button" />
<Button
android:id="@+id/button3"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onClick3"
android:text="Button" />
<Button
android:id="@+id/button4"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onClick4"
android:text="Button" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="onClick5"
android:text="Button" />
</LinearLayout>
</LinearLayout>
package com.example.app14;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.Bitmap.Config;
import android.graphics.BitmapFactory;
import android.graphics.Color;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
public class MainActivity extends Activity {
ImageView imageView;
Bitmap bitmap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = (ImageView) findViewById(R.id.imageView1);
bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.a);
imageView.setImageBitmap(bitmap);
}
public void onClick1(View view) {
Bitmap newBmp = bitmap.copy(Config.ARGB_8888, true);
for (int x = 0; x < newBmp.getWidth(); x++) {
for (int y = 0; y < newBmp.getHeight(); y++) {
int c = newBmp.getPixel(x, y);
int r = Color.red(c);
int g = Color.green(c);
int b = Color.blue(c);
int newColor = Color.argb(255, r, 0, 0);
newBmp.setPixel(x, y, newColor);
}
}
imageView.setImageBitmap(newBmp);
}
public void onClick2(View view) {
Bitmap newBmp = bitmap.copy(Config.ARGB_8888, true);
for (int x = 0; x < newBmp.getWidth(); x++) {
for (int y = 0; y < newBmp.getHeight(); y++) {
int c = newBmp.getPixel(x, y);
int r = Color.red(c);
int g = Color.green(c);
int b = Color.blue(c);
int gray = (r + g + b) / 3;
int newColor = Color.argb(255, gray, gray, gray);
newBmp.setPixel(x, y, newColor);
}
}
imageView.setImageBitmap(newBmp);
}
public void onClick3(View view) {
Bitmap newBmp = bitmap.copy(Config.ARGB_8888, true);
for (int x = 0; x < newBmp.getWidth(); x++) {
for (int y = 0; y < newBmp.getHeight(); y++) {
int c = newBmp.getPixel(x, y);
int r = 255 - Color.red(c);
int g = 255 - Color.green(c);
int b = 255 - Color.blue(c);
int newColor = Color.argb(255, r, g, b);
newBmp.setPixel(x, y, newColor);
}
}
imageView.setImageBitmap(newBmp);
}
public void onClick4(View view) {
Bitmap newBmp = bitmap.copy(Config.ARGB_8888, true);
for (int x = 0; x < newBmp.getWidth(); x++) {
for (int y = 0; y < newBmp.getHeight(); y++) {
int rgb = newBmp.getPixel(x, y);
int r = Color.red(rgb);
int g = Color.green(rgb);
int b = Color.blue(rgb);
int newR = (int) (0.393 * r + 0.769 * g + 0.189 * b);
int newG = (int) (0.349 * r + 0.686 * g + 0.168 * b);
int newB = (int) (0.272 * r + 0.534 * g + 0.131 * b);
int newColor = Color.argb(255, newR > 255 ? 255 : newR,
newG > 255 ? 255 : newG, newB > 255 ? 255 : newB);
newBmp.setPixel(x, y, newColor);
}
}
imageView.setImageBitmap(newBmp);
}
public void onClick5(View view) {
Bitmap newBmp = bitmap.copy(Config.ARGB_8888, true);
int x, y;
x = 100;
y = 100;
int newColor = 0;
for (int dx = x; dx < x + 200; dx++) {
for (int dy = y; dy < y + 200; dy++) {
if (dx % 50 == 0 || dy % 50 == 0) {
newColor = newBmp.getPixel(dx, dy);
} else {
newBmp.setPixel(dx, dy, newColor);
}
}
}
imageView.setImageBitmap(newBmp);
}
}