Handler定時數行應用
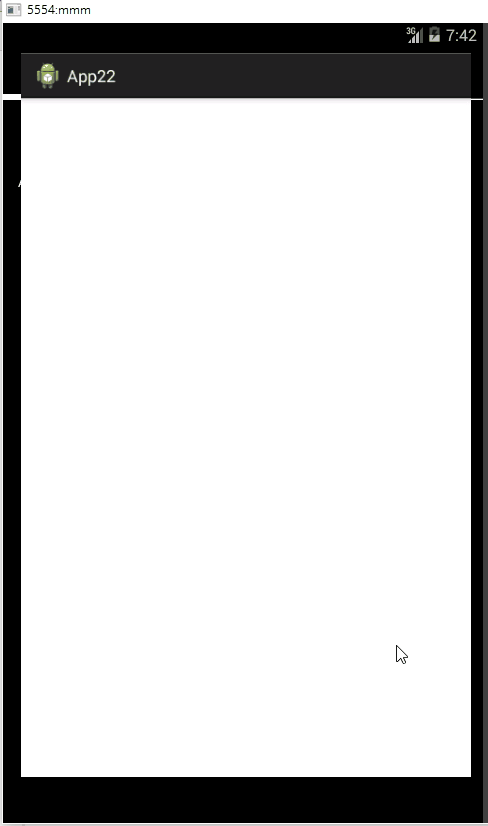
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/LinearLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="${relativePackage}.${activityClass}" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="matrix"
android:src="@drawable/ic_launcher" />
<ImageView
android:id="@+id/imageView5"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="fitXY"
android:src="@drawable/wall" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="matrix"
android:src="@drawable/ic_launcher" />
<ImageView
android:id="@+id/imageView6"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="fitXY"
android:src="@drawable/wall" />
<ImageView
android:id="@+id/imageView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="matrix"
android:src="@drawable/ic_launcher" />
<ImageView
android:id="@+id/imageView7"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="fitXY"
android:src="@drawable/wall" />
<ImageView
android:id="@+id/imageView4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="matrix"
android:src="@drawable/ic_launcher" />
<ImageView
android:id="@+id/imageView8"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="fitXY"
android:src="@drawable/wall" />
</LinearLayout>
package com.example.app22;
import android.app.Activity;
import android.content.res.Resources;
import android.graphics.Matrix;
import android.os.Bundle;
import android.os.Handler;
import android.util.Log;
import android.view.ViewTreeObserver.OnPreDrawListener;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends Activity {
Handler handler;
Resources res;
ImageView[] imageViews = new ImageView[4];
int[] len = new int[4];
int sw;
int iw;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
res = this.getResources();
for (int i = 0; i < imageViews.length; i++) {
int id = res.getIdentifier("imageView" + (i + 1), "id",
this.getPackageName());
imageViews[i] = (ImageView) this.findViewById(id);
}
handler = new Handler();
handler.postDelayed(updateTask, 2000);
}
@Override
public void onWindowFocusChanged(boolean hasFocus) {
if (hasFocus) {
sw = imageViews[0].getWidth();
iw = imageViews[0].getDrawable().getIntrinsicWidth();
Log.e("MainActivity", "width=" + sw);
}
super.onWindowFocusChanged(hasFocus);
}
Runnable updateTask = new Runnable() {
@Override
public void run() {
Log.e("MainActivity", "執行了");
if (race()) {
handler.postDelayed(updateTask, 200);
}
}
private boolean race() {
Matrix m = new Matrix();
for (int i = 0; i < imageViews.length; i++) {
int n = (int) (Math.random() * 5) + 5;
len[i] += n;
m.reset();
m.postTranslate(len[i], 0);
imageViews[i].setImageMatrix(m);
if (len[i] > sw - iw) {
String text = String.format("第%d隻贏了", i + 1);
Toast.makeText(MainActivity.this, text, Toast.LENGTH_SHORT)
.show();
return false;
}
}
return true;
}
};
@Override
protected void onDestroy() {
handler.removeCallbacks(updateTask);
super.onDestroy();
}
}